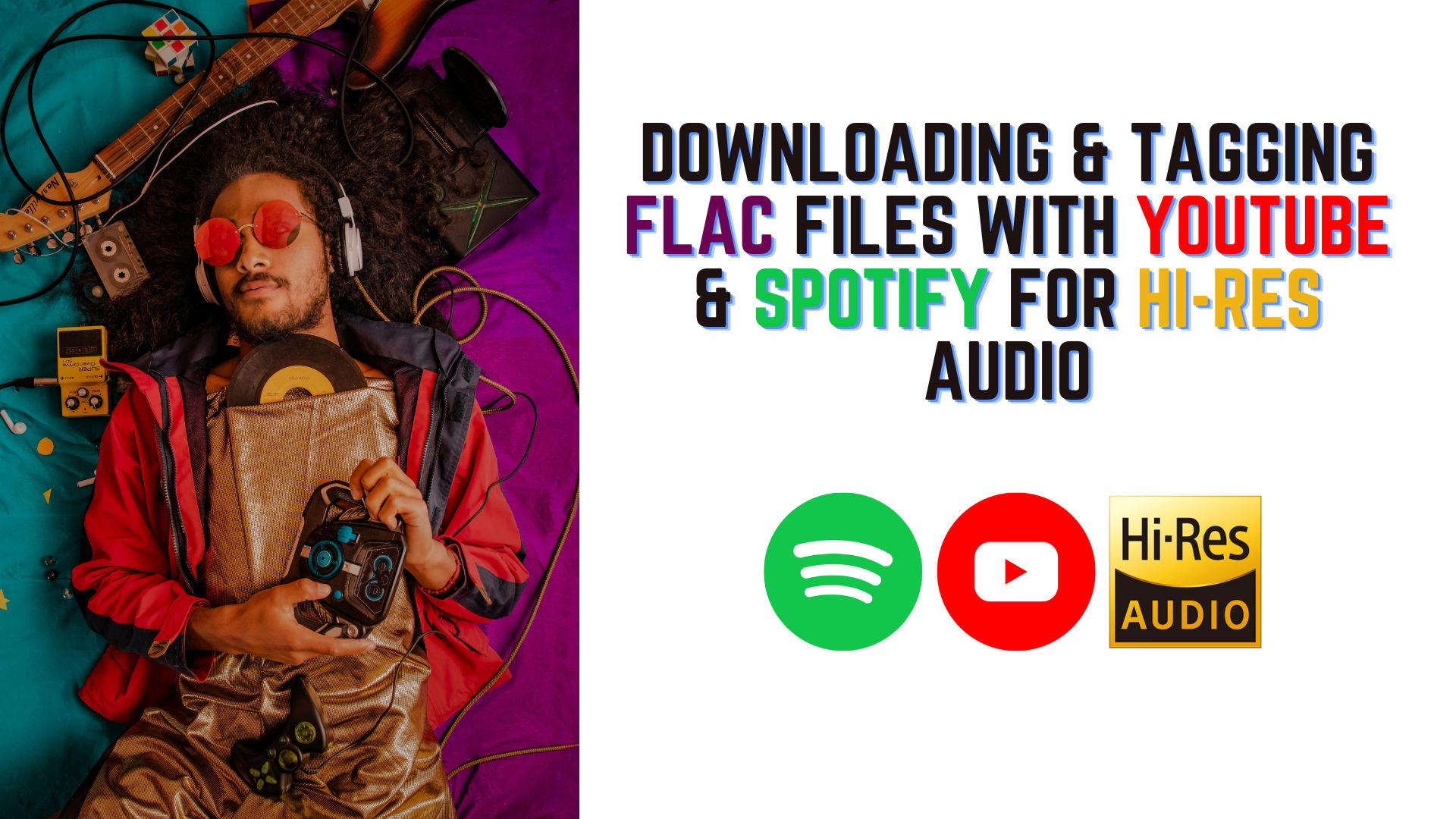
Exploring High-Resolution Audio: Downloading and Tagging FLAC Files with YouTube and Spotify
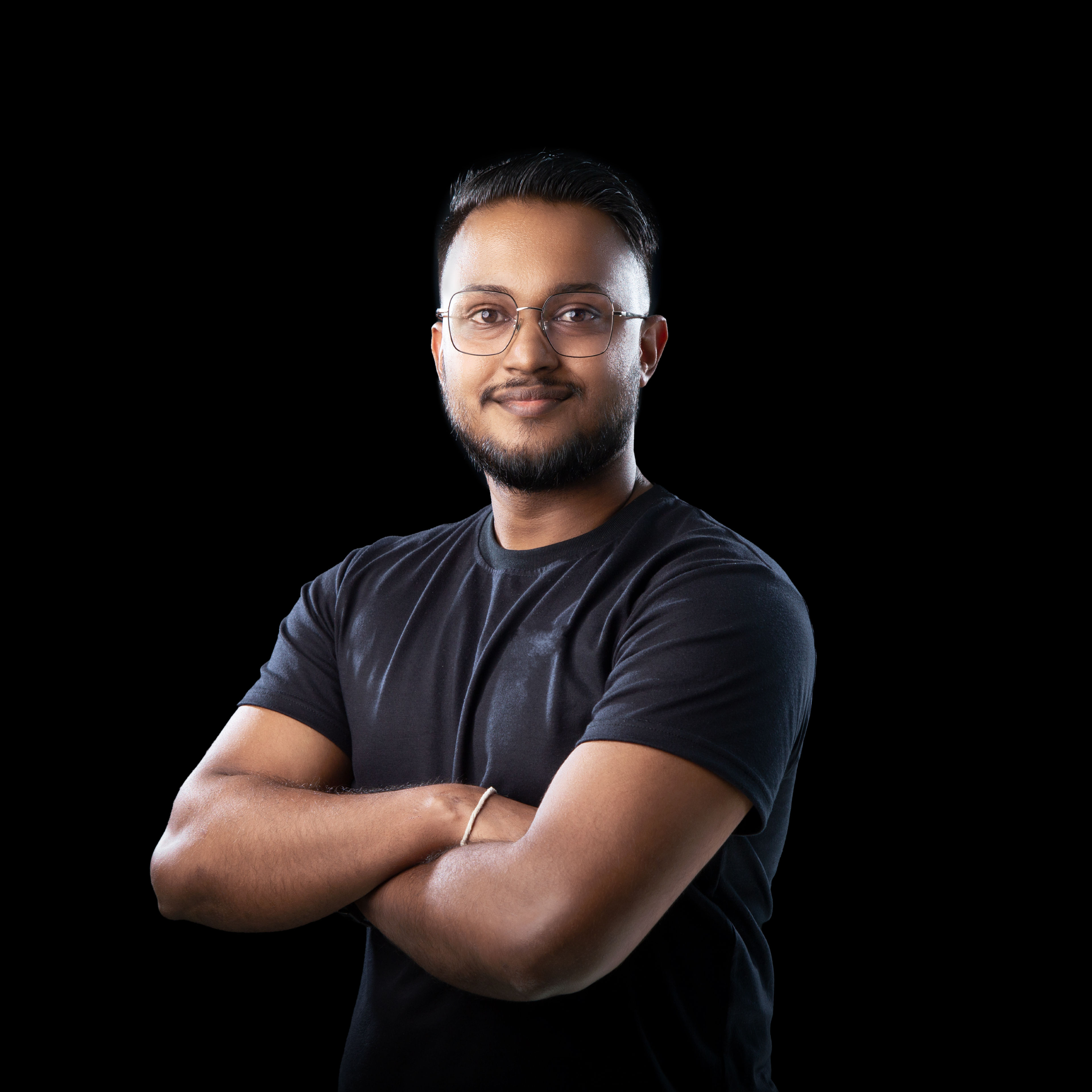
- June 17, 2024
- 6 min read
Introduction
In the era of streaming and digital music, audio quality often gets compromised for the sake of convenience. However, for audiophiles and music enthusiasts, high-resolution (hi-res) audio offers an unparalleled listening experience that captures the nuances and details of the original recordings. In this article, we'll explore what hi-res audio is, the importance of FLAC files, and how you can download and tag hi-res audio from YouTube using a Python script. This project is an experimental approach for anyone who loves to listen to hi-res audio without spending a fortune.
What is Hi-Res Audio?
High-resolution audio refers to music files that have a higher bit depth and sample rate than standard CD-quality audio. While CDs offer 16-bit/44.1kHz audio, hi-res audio can go up to 24-bit/192kHz. This higher resolution means more audio data, resulting in greater detail and clarity, closer to what the artists and producers intended.
Understanding FLAC Files
FLAC (Free Lossless Audio Codec) is a popular format for hi-res audio. Unlike MP3 or AAC, FLAC compresses audio without any loss of quality. This makes it ideal for preserving the full richness of hi-res audio recordings. FLAC files are larger in size compared to their lossy counterparts, but they offer superior audio fidelity, which is crucial for a true hi-res listening experience.
Why Listen to Hi-Res Audio?
Listening to hi-res audio offers several benefits:
- Enhanced Detail and Clarity: Hi-res audio captures subtleties and details that are often lost in compressed formats.
- Authentic Experience: It provides a listening experience that is closer to the original studio recording.
- Better Dynamics: Hi-res audio has greater dynamic range, allowing for a more immersive listening experience.
An Experimental Project: Downloading and Tagging Hi-Res Audio
To enjoy hi-res audio without breaking the bank, we can use a combination of YouTube, Spotify, and a Python script. This project involves downloading audio from YouTube in FLAC format and then tagging it with metadata from Spotify. Here’s how you can do it.
Step-by-Step Guide
Requirements
Before you start, ensure you have the following installed:
pip install yt-dlp pydub mutagen pillow requests spotipy
The Script
Here’s the Python script to download and tag hi-res audio:
import os
import glob
from pydub import AudioSegment
from mutagen.flac import FLAC, Picture
from PIL import Image
import requests
from yt_dlp import YoutubeDL
from spotipy.oauth2 import SpotifyOAuth
import spotipy
# Set up Spotify API credentials
SPOTIFY_CLIENT_ID = 'your_spotify_client_id'
SPOTIFY_CLIENT_SECRET = 'your_spotify_client_secret'
SPOTIFY_REDIRECT_URI = 'http://localhost:8888/callback'
credentials = SpotifyOAuth(client_id=SPOTIFY_CLIENT_ID, client_secret=SPOTIFY_CLIENT_SECRET, redirect_uri=SPOTIFY_REDIRECT_URI, scope="user-library-read")
sp = spotipy.Spotify(auth_manager=credentials)
def fetch_spotify_metadata(track_name):
results = sp.search(q=track_name, limit=1)
if results['tracks']['items']:
track = results['tracks']['items'][0]
album_cover_url = track['album']['images'][0]['url']
metadata = {
'title': track['name'],
'artist': track['artists'][0]['name'],
'album': track['album']['name'],
'album_cover_url': album_cover_url,
'release_date': track['album']['release_date']
}
return metadata
return None
def download_album_cover(url, output_path='album_cover.jpg'):
response = requests.get(url)
if response.status_code == 200:
with open(output_path, 'wb') as file:
file.write(response.content)
return output_path
return None
def add_metadata_to_flac(flac_file, metadata, album_cover_file):
audio = FLAC(flac_file)
audio['title'] = metadata['title']
audio['artist'] = metadata['artist']
audio['album'] = metadata['album']
audio['date'] = metadata['release_date']
image = Image.open(album_cover_file)
image_data = open(album_cover_file, 'rb').read()
picture = Picture()
picture.data = image_data
picture.type = 3 # Front cover
picture.mime = 'image/jpeg'
picture.width, picture.height = image.size
picture.depth = 24 # Color depth
audio.add_picture(picture)
audio.save()
def download_youtube_playlist(playlist_url, output_folder):
ydl_opts = {
'format': 'bestaudio/best',
'postprocessors': [{
'key': 'FFmpegExtractAudio',
'preferredcodec': 'flac',
'preferredquality': '0', # best quality
}],
'outtmpl': os.path.join(output_folder, '%(title)s.%(ext)s'),
}
with YoutubeDL(ydl_opts) as ydl:
ydl.download([playlist_url])
def process_audio_files(folder_path):
flac_files = glob.glob(os.path.join(folder_path, '*.flac'))
for flac_file in flac_files:
track_name = os.path.basename(flac_file).replace('.flac', '')
metadata = fetch_spotify_metadata(track_name)
if metadata:
album_cover_file = download_album_cover(metadata['album_cover_url'])
if album_cover_file:
add_metadata_to_flac(flac_file, metadata, album_cover_file)
os.remove(album_cover_file) # Clean up album cover file
# Example usage
playlist_url = 'YOUR_YOUTUBE_PLAYLIST_URL' # Replace with your YouTube playlist URL
folder_path = '.' # Update this path if needed
# Step 1: Download YouTube playlist
download_youtube_playlist(playlist_url, folder_path)
# Step 2: Add metadata
process_audio_files(folder_path)
Sample YouTube Playlist: https://www.youtube.com/watch?v=kPBzTxZQG5Q&list=PL6Ve5Ag9Wqe5xf7gR2KCF6RCc8ALFeQDu
Run the Script
After saving the script to a file as a Python script, run it using the command below:
python songchanger.py
Explanation
- Download YouTube Playlist:
- The
download_youtube_playlist
function usesyt-dlp
to download audio from each video in the playlist in FLAC format, ensuring the highest quality. - The
yt_dlp.YoutubeDL
class is configured with options to download the best audio and convert it to FLAC.
- The
- Add Metadata:
- The
process_audio_files
function handles adding metadata and album art to the FLAC files usingmutagen
.
- The
Conclusion
High-resolution audio provides an exceptional listening experience by preserving the intricate details of the original recordings. Using the provided Python script, you can download hi-res audio from YouTube and enrich it with metadata from Spotify, making it a cost-effective way to enjoy your favorite music in superior quality. Whether you're a casual listener or a dedicated audiophile, this project offers a practical way to elevate your audio experience.
Try out this script and transform your music library into a hi-res audio collection. Share your experiences and any improvements you make to the script in the comments below. Happy listening!
hi-res audio
FLAC
Python
yt-dlp
Spotify
audiophile
music